After I had written most of this article, I realized that I may have come up with a subject that interests absolutely no one but me. You see, this is about knitting like a programmer. How many of you both program, and knit? I mentioned this problem to Bart and he explained that there’s a long history of the intersection of programming and textiles. Bart went on to explain that programming punch cards have their origins in weaving.
He pointed me to a Wikipedia article about the Jacquard machine, which was designed to program a loom to automate the process of weaving. From the Wikipedia article about the Jacquard machine:
The machine was controlled by a “chain of cards”; a number of punched cards laced together into a continuous sequence. Multiple rows of holes were punched on each card, with one complete card corresponding to one row of the design.
So maybe this intersection of programming and knitting will be of some interest after all.
I’ve been programming for a few years now, but I’ve been knitting since I was very young. I actually taught myself to knit when I was around 9 years old, learning from a book. I can distinctly remember learning the stockinette stitch at the kitchen table and being SO frustrated until I figured out that the dumb instructions didn’t tell you to move the yarn from back to front to back as you switch from knit to purl and back again. Once I figured that out it was smooth sailing.
I haven’t knit for a very long time, keeping my fingers busy with crocheting and cross-stitching for at least the last 25 years. But my daughter-in-law Nikki chose a knitted pattern for the baby blanket I’m making for my future grandson. It was time to get back into the game.
The Problem to be Solved
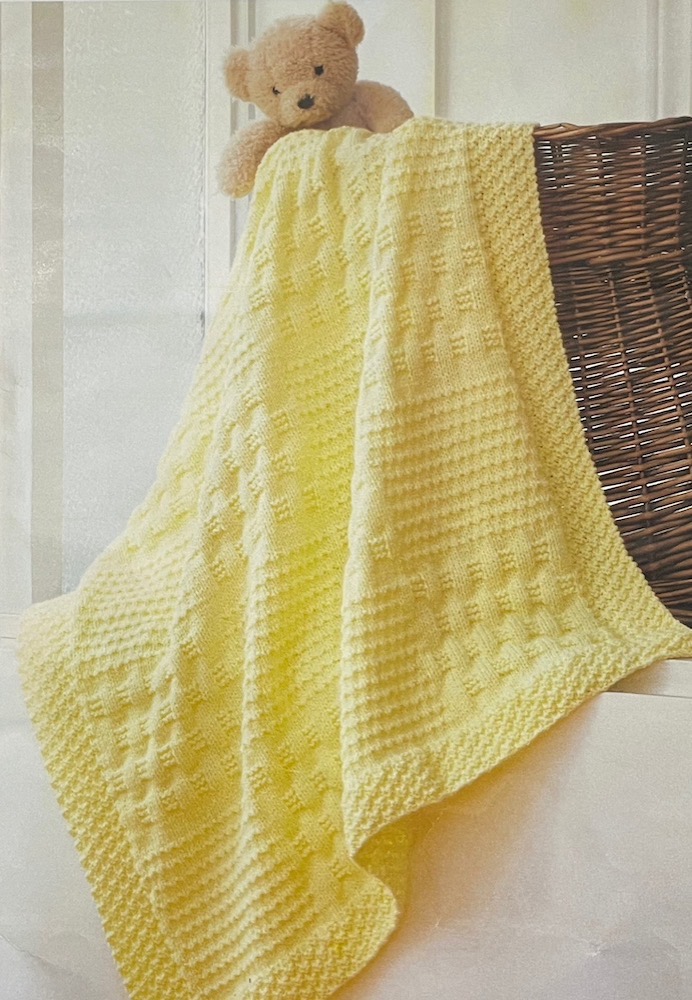
Execution of some knitting patterns wouldn’t benefit from thinking like a programmer, but the particular pattern I’m working on cries out for it. Knitting patterns (and crochet patterns for that matter) will give you row by row instructions, but whenever a complex bit is repeated, they’ll often throw an asterisk on either side and say “repeat from * to *”.
In programming, if you’re going to repeat something, you make it a function so you can repeat it predictably. Already we can see that knitting and programming have something in common.
The problem I was trying to solve was that this pattern is written in a very complicated way. This resulted in constant mistakes on my part until I started to break the pattern down in a different way.
If you look at the photo on the cover of the instructions, you see an alternating pattern of big square blocks. Every other big square block is either checked or striped, and there are four big rows of blocks. The instructions call these big rows of blocks “sets”. So on the first set, it’s blocks of check/stripe/check/stripe, but on set two it’s stripe/check/stripe/check.
That photo is critical to figuring out the pattern, because down in the weeds of the instructions, it’s very difficult to see this big picture view I’ve described.
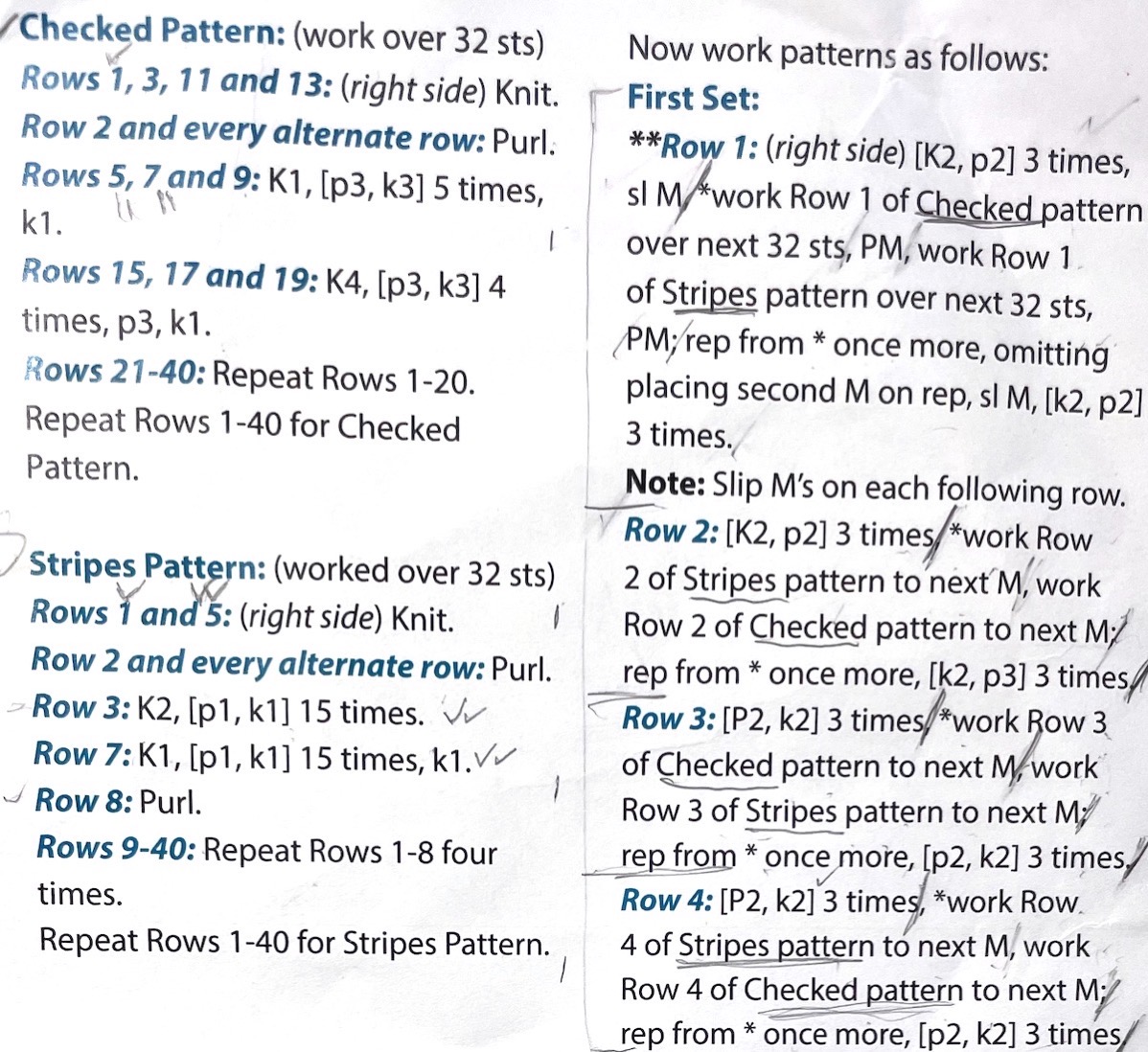
The pattern describes the sets in a very complex way. Let’s say you’re on row 26.
Row 26 isn’t explicitly explained. It says that row 26 is row 21 in the check pattern. Ok, what’s row 21? Well, row 21 is a repeat of row 1 in the check pattern. I’m not making this up.
I started by creating two lookup tables but it was like doing two coordinate transformations in your head! I tore out many rows many times.
That’s when I started to think of it as functions and it became much clearer to me.
Let’s think of the check and stripe blocks as functions, because inside each of those blocks, we have a set of instructions. We’re going to call them stripeBlock and checkBlock.
function stripeBlock() {do stripe-type stuff}
function checkBlock() {do check-type stuff}
StripeBlock
Now let’s dig down inside our two functions. The instructions for the stripeBlock function defines explicitly what to do on rows 1-8. All of the even rows (2, 4, 6, and 8) are a simple purl stitch all the way across.
So we could think of the purl rows (the even ones) as their own function, which we’ll simply call purl.
function purl() {purl}
Rows 1 and 5 are both a simple knit stitch, so we’ll define a knit function for those rows.
function knit() {knit}
That leaves rows 3 and 7 for the interesting functions. Both rows 3 and 7 are what creates the look of the stripe in the pattern. It’s what I’d call a bumpy-looking row created by alternating knit and purl. But to make it a little more interesting, rows 3 and 7 are offset from each other so the bumps don’t line up. For that reason, we have to define two functions, which we’ll call stripe3 and stripe7. In the function stripe3, we’re going to knit 2, but then 15 times we will purl 1, knit 1. Well, that sounds like a for loop in programming to me!
function stripe3() {
k2;
for (i=1; i<16; i++){ // e.g. repeat 15X
p1,k1;
};
};
The stripe7 function is just slightly different so that we get the offset I was talking about. Instead of starting with knit 2, it starts with knit 1 and ends with a knit 1, but it has the same for loop inside. If I was refactoring this to clean it up, I’d probably define our little for loop of p1, k1 15 times as yet another function.
function stripe7() {
k1;
for (i=1; i<16; i++){ // e.g. repeat 15X
p1,k1
};
k1;
};
Now the reason it’s helpful to think of this knitting pattern as this series of functions is that this group of 8 rows gets repeated 5 times for a total of 40 rows in one stripeBlock.
Putting the stripeBlock function together then, we need to repeat our pattern of 1 to 8, five times.
function stripeBlock(){
for (j=1; j<6; j++){ // repeat 5x
for (k=1; k<9; k++){ // 8 rows
if (i=even){ // all even rows
purl();
};
if (i=1 | i=5){ // just rows 1 and 5
knit();
};
if (i=3){ // just row 3
stripe3();
};
if (i=7){ // just row 7
stripe7();
};
};
};
};
How about that checkBlock?
This would be a great stopping point if the checkBlock repeated at the same rate internally as the stripeBlock, but nooooo, the inventor of this pattern made them repeat at different rates.
While the stripeBlock function cycles through every 8 rows, the checkBlock cycles every 20 rows! Now, this wouldn’t be so bad if the two blocks were independent, but they’re not. Remember we’re going across and making a stripeBlock then a checkBlock then a stripeBlock then a checkBlock.
That means that at 8 rows we have to start our repeat over again for the stripeBlock, but we don’t yet change the checkBlocks. At 20 rows though, the checkBlocks start their repeat, but the stripeBlocks don’t change. It’s really really hard to keep track!
I know you’re really hoping that I’ll go through the pseudo-code for the checkBlock but for brevity, I’ll just put it in the shownotes.
function check1() {
k1;
for (i=1; i<6; i++){ // repeat 5X
p3,k3;
};
k1;
};
function check2() {
k4;
for (i=1; i<5; i++){ // repeat 4X
p3,k3;
};
p3,k1;
};
function checkBlock(){
for (j=1; j<6; j++){ // repeat 5x
for (k=1; k<20; k++){ // 20 rows
if (i=even){ // all even rows
purl();
};
if (i=1 | i=3 | i=11 | i=13){ // rows 1, 3, 11, and 13
check1;
};
if (i=15 | i=17 | i=19){ // rows 15, 17 and 19
check2;
};
};
};
Understanding this pattern at this fundamental level was critical to being able to even tell if I’d messed up. But it doesn’t help me actually knit each row. I can’t run these functions. I have to still create every stitch one at a time.
I pulled out one of my favorite tools when working on crafts: Notability. I wrote out all 40 rows of this first set by hand using the Apple Pencil on my iPad Pro.
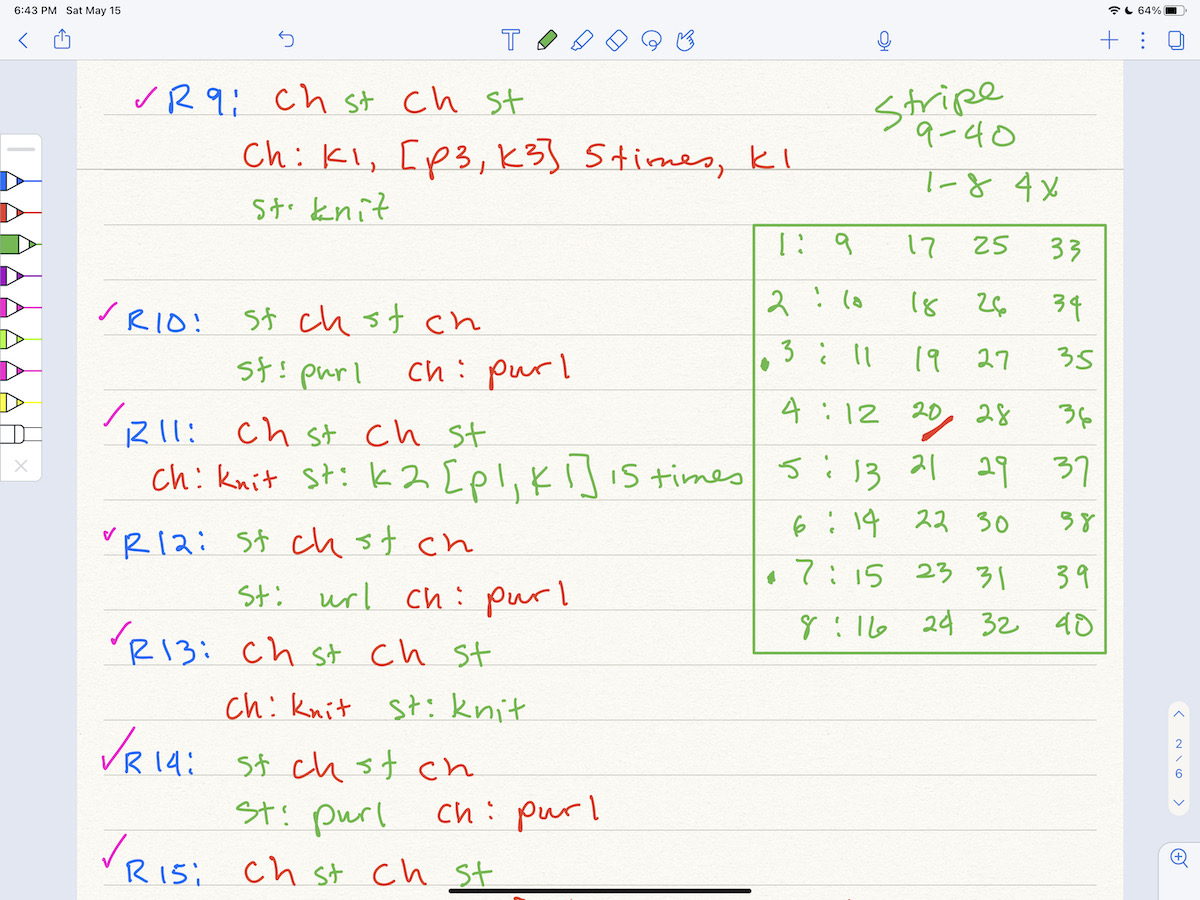
I wrote the stripe instructions in green for each row and the check instructions in red. That turned out to be invaluable in figuring out where I was. Since I’d figured out the pattern in a programming way, once I had 20 row numbers written out, I could copy and paste between rows to reduce errors. For example, in the checkBlock, rows 1, 3, 11, and 13 are the same so I could just write it out once, copy, and paste it three times.
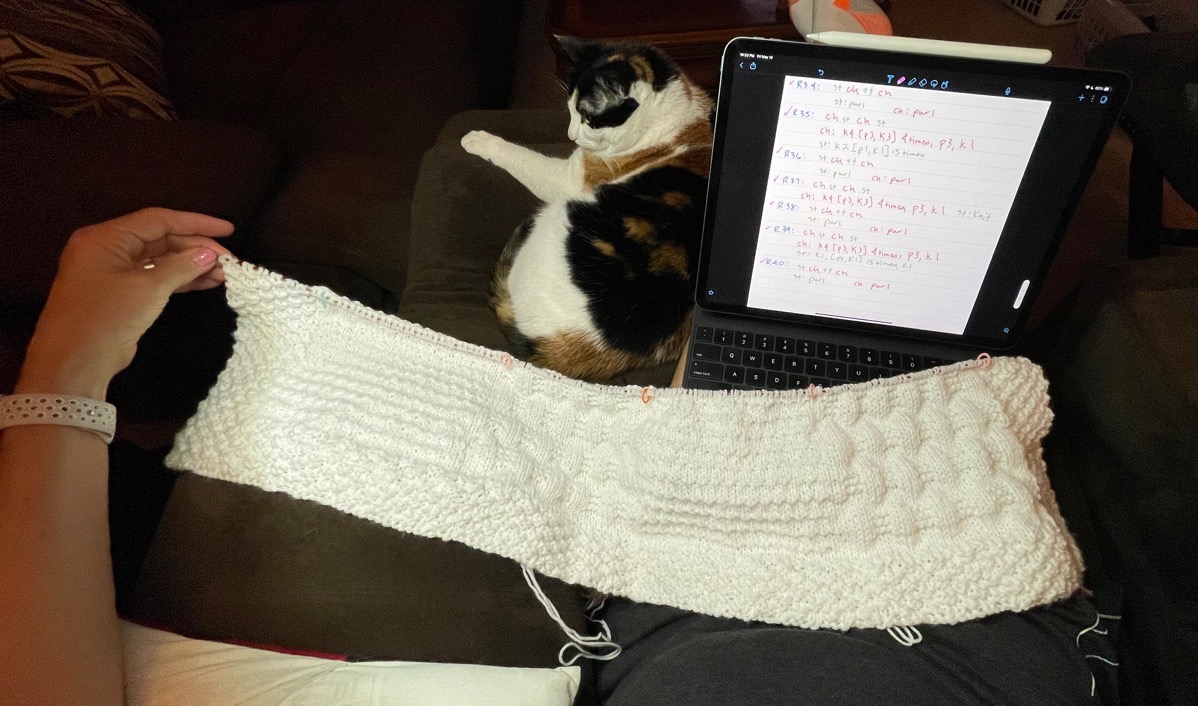
Once I had the 40 rows written out for the first set of four blocks, I could finally start knitting the pattern predictably. I sit in my easy chair with my iPad Pro in the Magic Keyboard as a stand. I open to Notability while I knit, and use the Apple Pencil to check off each row. On a few occasions I’ve been knitting in the car, and the iPhone is a more practical tool for that environment. Notability works on both platforms (and the Mac) and syncs notes perfectly. I can zoom way up on Notability on iPhone and use my big, fat fingers to make my little checkmarks and it works surprisingly well.
I’m not going to pretend that I’m not making mistakes with this process, mind you, but I have a much better feel for the pattern with the process I followed.
I’ve just finished the first 40 rows, and that means I get to start set 2. Set 1 was stripe/check/stripe/check, and set 2 will be check/stripe/check/stripe. You would think that I could just grab the blocks and swap them, but I can’t. I’m going to have to write out every single row by hand again because of that darn cycling by 8 rows vs. cycling by 20 rows.
Bottom line
The bottom line is that it baffles me how “normal” people knit. This pattern is rated “Easy”, and yet I find it very complicated. My suspicion is that the difficulty rating is weighted more heavily by the difficulty of the stitches themselves, rather than the entire pattern as a whole. This only has knit and purl in it, no cable stitches, no ribbing, no stockinette, so I’m thinking that’s why they think it is easy.
I’m having fun making the blanket, and while the journey in this kind of craft is half the enjoyment, I can’t wait to wrap it around my new grandson when he makes his arrival.
Very interesting exercise in repeated processes. I remember, (more than) a few years ago, that you said you were not a programmer. But now… Fun isn’t it?
116 episodes of Programming by Stealth and I think I am a programmer now. Weird to say it out loud but I’ve written a real web app that solves a real problem and several people find it useful! I am loving it, just wish I could carve out more time to code. The podcasts take a LOT of time as it turns out!
Ada, Countess of Lovelace, arguably the world’s first programmer, opined “The Analytical Engine weaves algebraic patterns, just as the Jacquard loom weaves flowers and leaves.” see https://www.scienceandindustrymuseum.org.uk/objects-and-stories/jacquard-loom#inspiring-early-computing
Sarah and I got to see a replica of a Difference Engine at the London Science Museum and those punch cards
So maybe Ada and I would both enjoy this story then?
Indeed! As long as she doesn’t unwind every bit of the worsted (https://en.wikipedia.org/wiki/Worsted and “Through the Looking Glass” )
So happy to read your comment ‘I am a programmer now’ — I am also older and learning to code and started PBS after listening to (and enjoying) your Code Newbie chat. I’m up to Episode 17 and I really wanted to know if you become a programmer in the end, lol. I can’t wait to say the same sentence. I am sure if I keep up with the podcast I will be able to say it too!! Thanks for taking us along on your journey! Happy coding and knitting and snuggling with your grandbaby!
I love this message, Kim! Yes, I’m a programmer, which is still hard to say out loud because I feel like a pretender, but everyone tells me that PROVES I’m a programmer. Evidently imposter syndrome comes with the title.
Here’s a couple of posts you might like at this stage in your coding journey. This one was inspired by an episode of Code Newbie – I wrote out what it feels like, the struggle, the triumph, the struggle.
https://www.podfeet.com/blog/2020/06/real-developer/
In this one I explained how Programming is my happy place:
https://www.podfeet.com/blog/2020/04/programming-happy-place/
And after using a lot of different code editors, I settled on Visual Studio Code by Microsoft. I wrote up why from a beginner developer’s perspective. It’s fantastic for the very advanced but works really well for the less advanced.
https://www.podfeet.com/blog/2020/10/vscode-beginner/
Good luck on your journey and keep me posted how you get along. We have a Slack group for all of my podcast work and it has a dedicated Programming by Stealth channel where really nice people are very willing to help out. Check it out at https://podfeet.com/slack.